mirror of
https://github.com/codewriter-packages/Tri-Inspector.git
synced 2025-01-22 08:18:49 -05:00
Readme
This commit is contained in:
parent
9dd4c9e9df
commit
5abfc75a89
74
README.md
74
README.md
@ -24,17 +24,19 @@ _Advanced inspector attributes for Unity_
|
||||
|
||||
Shows non-serialized property in the inspector.
|
||||
|
||||

|
||||
|
||||
```csharp
|
||||
private float field;
|
||||
private float _field;
|
||||
|
||||
[ShowInInspector]
|
||||
public float ReadOnlyProperty => field;
|
||||
public float ReadOnlyProperty => _field;
|
||||
|
||||
[ShowInInspector]
|
||||
public float EditableProperty
|
||||
{
|
||||
get => field;
|
||||
set => field = value;
|
||||
get => _field;
|
||||
set => _field = value;
|
||||
}
|
||||
```
|
||||
|
||||
@ -42,16 +44,24 @@ public float EditableProperty
|
||||
|
||||
Changes property order in the inspector.
|
||||
|
||||

|
||||
|
||||
```csharp
|
||||
[PropertyOrder(1)]
|
||||
public float first;
|
||||
|
||||
[PropertyOrder(0)]
|
||||
public float second;
|
||||
```
|
||||
|
||||
#### ReadOnly
|
||||
|
||||
Makes property non-editable.
|
||||
Makes property non-editable in the inspector.
|
||||
|
||||

|
||||
|
||||
```csharp
|
||||
[ReadOnly]
|
||||
public Vector3 vec;
|
||||
```
|
||||
|
||||
#### OnValueChanged
|
||||
@ -74,37 +84,40 @@ Tri Inspector has some builtin validators such as `missing reference` and `type
|
||||
Additionally you can mark out your code with validation attributes
|
||||
or even write own validators.
|
||||
|
||||

|
||||
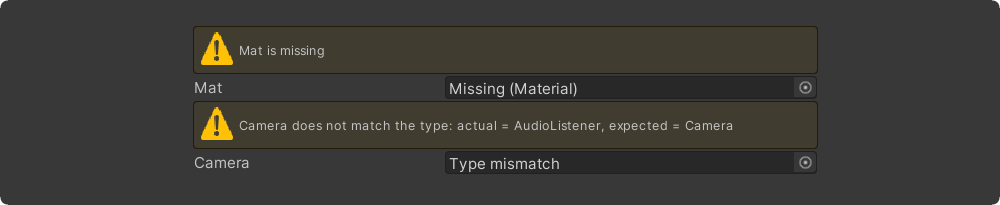
|
||||
|
||||
#### Required
|
||||
|
||||

|
||||
|
||||
```csharp
|
||||
[Required]
|
||||
public Material mat;
|
||||
```
|
||||
|
||||

|
||||
|
||||
#### ValidateInput
|
||||
|
||||

|
||||
|
||||
```csharp
|
||||
[ValidateInput(nameof(ValidateTexture))]
|
||||
public Textute tex;
|
||||
public Texture tex;
|
||||
|
||||
private TriValidationResult ValidateTexture()
|
||||
{
|
||||
if (tex == null) return TriValidationResult.Error("Tex is null");
|
||||
if (!tex.isReadable) return TriValidationResult.Warning("Tex must be readable");
|
||||
return TriValidationResult.Valid;
|
||||
}
|
||||
|
||||
```
|
||||
|
||||

|
||||
|
||||
### Styling
|
||||
|
||||
#### HideLabel
|
||||
```csharp
|
||||
[HideLabel]
|
||||
```
|
||||

|
||||
|
||||
#### LabelText
|
||||
```csharp
|
||||
@ -137,8 +150,6 @@ private TriValidationResult ValidateTexture()
|
||||
public int val;
|
||||
```
|
||||
|
||||

|
||||
|
||||
#### Header
|
||||
```csharp
|
||||
[Header("My Header")]
|
||||
@ -156,14 +167,18 @@ public int val;
|
||||
```
|
||||
|
||||
#### InlineEditor
|
||||
|
||||
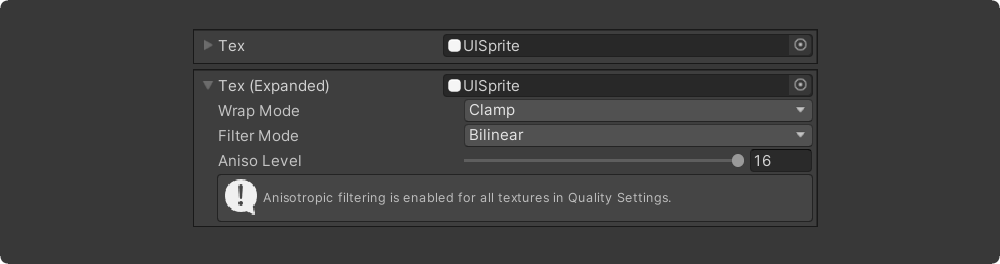
|
||||
|
||||
```csharp
|
||||
[InlineEditor]
|
||||
public Material mat;
|
||||
```
|
||||
|
||||
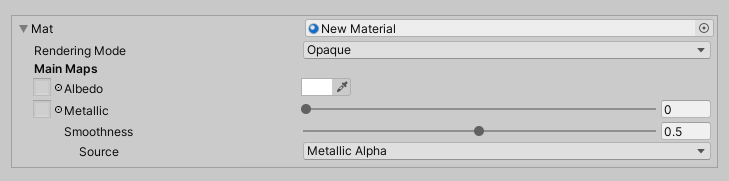
|
||||
|
||||
#### InlineProperty
|
||||
|
||||

|
||||
|
||||
```csharp
|
||||
public MinMax rangeFoldout;
|
||||
|
||||
@ -178,20 +193,20 @@ public class MinMax
|
||||
}
|
||||
```
|
||||
|
||||

|
||||
|
||||
### Collections
|
||||
|
||||
#### ListDrawerSettings
|
||||
|
||||
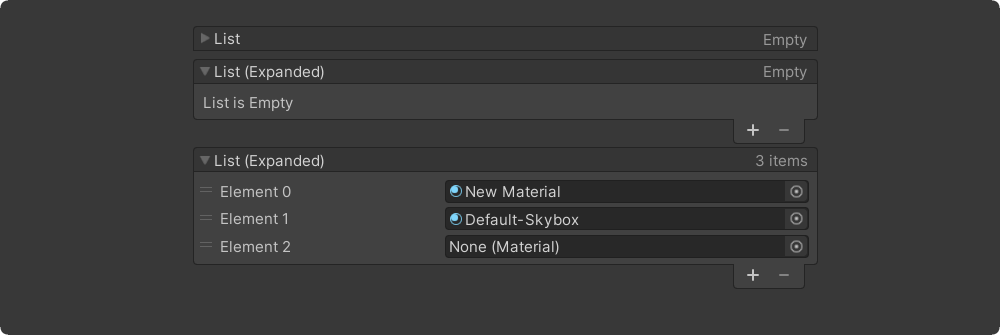
|
||||
|
||||
```csharp
|
||||
[ListDrawerSettings(Draggable = true,
|
||||
HideAddButton = false,
|
||||
HideRemoveButton = false,
|
||||
AlwaysExpanded = false)]
|
||||
public List<Material> list;
|
||||
```
|
||||
|
||||

|
||||
|
||||
### Conditionals
|
||||
|
||||
#### ShowIf
|
||||
@ -250,16 +265,17 @@ public float val;
|
||||
### Buttons
|
||||
|
||||
#### Button
|
||||
|
||||

|
||||
|
||||
```csharp
|
||||
[Button("My Button")]
|
||||
[Button("Click me!")]
|
||||
private void DoButton()
|
||||
{
|
||||
Debug.Log("Button clicked!");
|
||||
}
|
||||
```
|
||||
|
||||

|
||||
|
||||
### Debug
|
||||
|
||||
#### ShowDrawerChain
|
||||
@ -269,6 +285,8 @@ private void DoButton()
|
||||
|
||||
### Groups
|
||||
|
||||
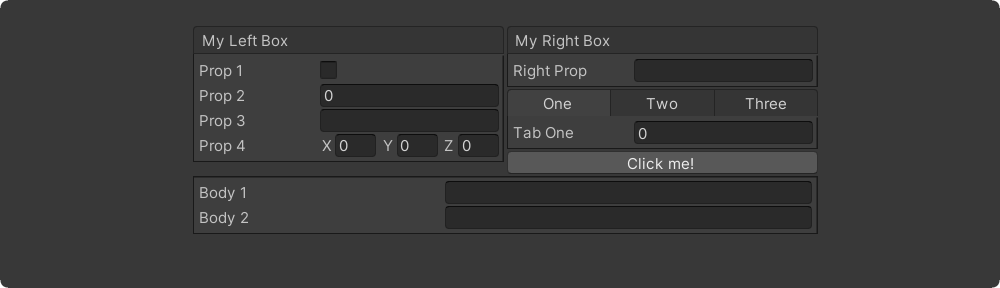
|
||||
|
||||
```csharp
|
||||
[DeclareHorizontalGroup("header")]
|
||||
[DeclareBoxGroup("header/left", Title = "My Left Box")]
|
||||
@ -292,15 +310,13 @@ public class GroupDemo : MonoBehaviour
|
||||
[Group("header/right/tabs"), Tab("Two")] public float tabTwo;
|
||||
[Group("header/right/tabs"), Tab("Three")] public float tabThree;
|
||||
|
||||
[Group("header/right"), Button]
|
||||
[Group("header/right"), Button("Click me!")]
|
||||
public void MyButton()
|
||||
{
|
||||
}
|
||||
}
|
||||
```
|
||||
|
||||
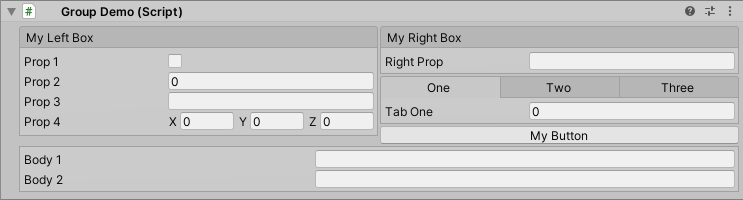
|
||||
|
||||
### Customization
|
||||
|
||||
#### Custom Drawers
|
||||
@ -467,7 +483,9 @@ Minimal Unity Version is 2020.3.
|
||||
Library distributed as git package ([How to install package from git URL](https://docs.unity3d.com/Manual/upm-ui-giturl.html))
|
||||
<br>Git URL: `https://github.com/codewriter-packages/Tri-Inspector.git`
|
||||
|
||||
After installing the package, you need to unpack the `Installer.unitypackage` that comes with the package
|
||||
After installing the package, you need to unpack the `Installer.unitypackage` that comes with the package.
|
||||
|
||||
Then in `ProjectSettings`/`TriInspector` enable `Full` mode for Tri Inspector.
|
||||
|
||||
## License
|
||||
|
||||
|
Loading…
Reference in New Issue
Block a user